Python
Unlocking the Power of Python Programming
At AdviTech IT Services, we offer a comprehensive Python course designed to equip you with the essential skills for programming, automation, data analysis, and web development. Our expert instructors guide you through Python’s core concepts, from basic syntax to advanced techniques like object-oriented programming and data manipulation. Through hands-on projects, real-world applications, and problem-solving exercises, you’ll gain practical experience and confidence in coding. With flexible schedules and personalized learning support, AdviTech IT Services ensures you master Python at your own pace, preparing you for a successful career in the tech industry.
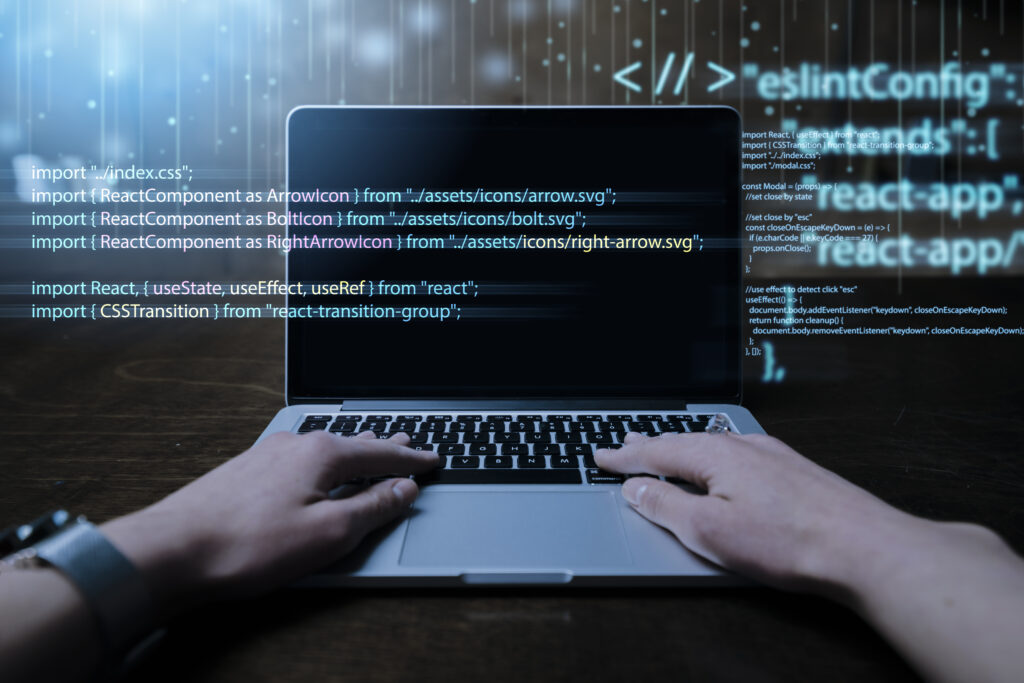
Why Choose Us?
- Expert Instructors: Learn from experienced Python developers with deep industry knowledge and practical experience.
- Comprehensive Curriculum: Master Python programming, covering everything from basic syntax to advanced topics like web development and data analysis.
- Hands-On Projects: Work on real-world projects and coding challenges to apply your skills in practical scenarios.
- Flexible Learning Options: Choose from a range of class timings, including weekend and evening batches, to fit your schedule.
- Career-Oriented Training: Focus on developing Python skills that are in high demand across various industries, including software development, automation, and data science.
- Placement Assistance: Receive support in connecting with top companies for job placements, helping you kickstart your career in Python.
- State-of-the-Art Facilities: Access modern tools, software, and infrastructure to enhance your learning experience.
- Certification: Earn a recognized Python certification to validate your skills and improve your job prospects.
- Global Alumni Network: Join a network of successful professionals who have excelled in their careers after training with AdviTech IT Services.
Course Content
Python
1. Python Introduction [7 hours]
- Getting started [1 hour theory/practical]
- Keywords and Identifier [1 hour theory/practical]
- Statements & Comments [1 hour theory/practical]
- Python Data types [1 hour theory/practical]
- Python Operators [2 hour theory/practical]
- Python I/O and Import [1 hour theory/practical]
- Practical
2. Python Native Data types [8 hours]
- Python Numbers [1 hour theory/practical]
- Python Strings [2 hour theory/practical]
- Python List [2 hour theory/practical]
- Python Tuple [1 hour theory/practical]
- Python Set [1 hour theory/practical]
- Python Dictionary [1 hour theory/practical]
- Practical
3. Python Flow Control Statements [7 hours]
- Python if…else [2 hour theory/practical]
- Python for Loop [1 hour theory/practical]
- Python while Loop [1 hour theory/practical]
- Python break and continue [1 hour theory/practical]
- Python Match Case. [1 hour theory/practical]
- Python Pass [1 hour theory/practical]
- Practical
4. Python Functions [8 hours]
- Python Function [1 hour theory/practical]
- Function Argument [1 hour theory/practical]
- Anonymous Function [1 hour theory/practical]
- Python Recursion [1 hour theory/practical]
- Higher Order Function [1 hour theory/practical]
- Variable Scope in Function [1 hour theory/practical]
- Python Modules [1 hour theory/practical]
- Python Package [1 hour theory/practical]
- Practical
5. Python Files [5 hours]
- Python File Operation [1 hour theory/practical]
- Python Directory [1 hour theory/practical]
- Python Exception [1 hour theory/practical]
- Exception handling [1 hour theory/practical]
- User-defined Exception [1 hour theory/practical]
- Practical
6. Python Object & Class [9 hours]
- Python Namespace [1 hour theory/practical]
- Python Class & Object [1 hour theory/practical]
- Python Encapsulation [1 hour theory/practical]
- Python Abstraction [1 hour theory/practical]
- Python Polymorphism [2 hour theory/practical]
- Python Inheritance [3 hour theory/practical]
- Practical
7. Advanced Topics [6 hours]
- Python Iterator [1 hour theory/practical]
- Python Generator [1 hour theory/practical]
- Python Closure [1 hour theory/practical]
- Python Decorators [1 hour theory/practical]
- Python Property [1 hour theory/practical]
- Python Examples [1 hour theory/practical]
- Practical
8. Python Programing Example
- Introduction
- Python Program to Print Hello world!
- Python Program to Add Two Numbers.
- Python Program to Find the Square Root.
- Python Program to Calculate the Area of a Triangle.
- Python Program to Solve Quadratic Equation.
- Python Program to Swap Two Variables.
- Python Program to Generate a Random Number.
- Python Program to Convert Kilometers to Miles.
- Python Program to Convert Celsius To Fahrenheit.
- Decision Making loops
- Python program to check if a number is positive, negative or zero.
- Python Program to Check if a Number is Odd or Even.
- Python Program to Check Leap Year.
- Python Program to Find the Largest Among Three Numbers.
- Python Program to Check Prime Number.
- Python Program to Print all Prime Numbers in an Interval.
- Python Program to Find the Factorial of a Number.
- Python Program to Display the multiplication Table.
- Python Program to Print the Fibonacci sequence.
- Python Program to Check Armstrong Number.
- Python Program to Find Armstrong Number in an Interval.
- Python Program to Find the Sum of Natural Numbers.
- Function
- Python Program To Display Powers of 2 Using Anonymous Function.
- Python Program to Find Numbers Divisible by Another Number.
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal.
- Python Program to Find ASCII Value of Character.
- Python Program to Find HCF or GCD.
- Python Program to Find LCM.
- Python Program to Find Factors of Number.
- Python Program to Make a Simple Calculator.
- Python Program to Shuffle Deck of Cards.
- Python Program to Display Calendar.
- Python Program to Display Fibonacci Sequence Using Recursion.
- Python Program to Find Sum of Natural Numbers Using Recursion.
- Native Data type
- Python Program to Add Two Matrices.
- Python Program to Transpose a Matrix.
- Python Program to Multiply Two Matrices.
- Python Program to check whether a String is Palindrome or Not.
- Python Program to Remove Punctuations From a String.
- Python Program to Sort Words in Alphabetic Order.
- Python Program to Illustrate Different Set Operations.
- Python Program to Count the Number of Each Vowel.
- File
- Python Program to Merge Mails.
- Python Program to Find the Size (Resolution) of an Image.
- Python Program to Find Hash of File.
9. Python MySQL Connectivity
- Getting started with MySQL python connector
- Python connecting to MySQL databases
- Python MySQL query
- Python create Table
- Python MySQL insert data
- Python MySQL retrieve data
- Python MySQL update data
- Python MySQL delete data