Data Structure
AdviTech IT Services: Navigating the Core of Data Structures
At AdviTech IT Services, we offer a comprehensive Data Structure course designed to build a strong foundation for efficient programming and problem-solving. Our expert instructors deliver in-depth lessons on core concepts like arrays, linked lists, stacks, queues, trees, and graphs, ensuring you understand their practical applications. Through hands-on projects and real-world scenarios, you’ll learn how to optimize code and solve complex problems effectively. With flexible learning schedules, personalized support, and career-focused training, AdviTech IT Services equips you with the skills needed to excel in competitive technical roles.
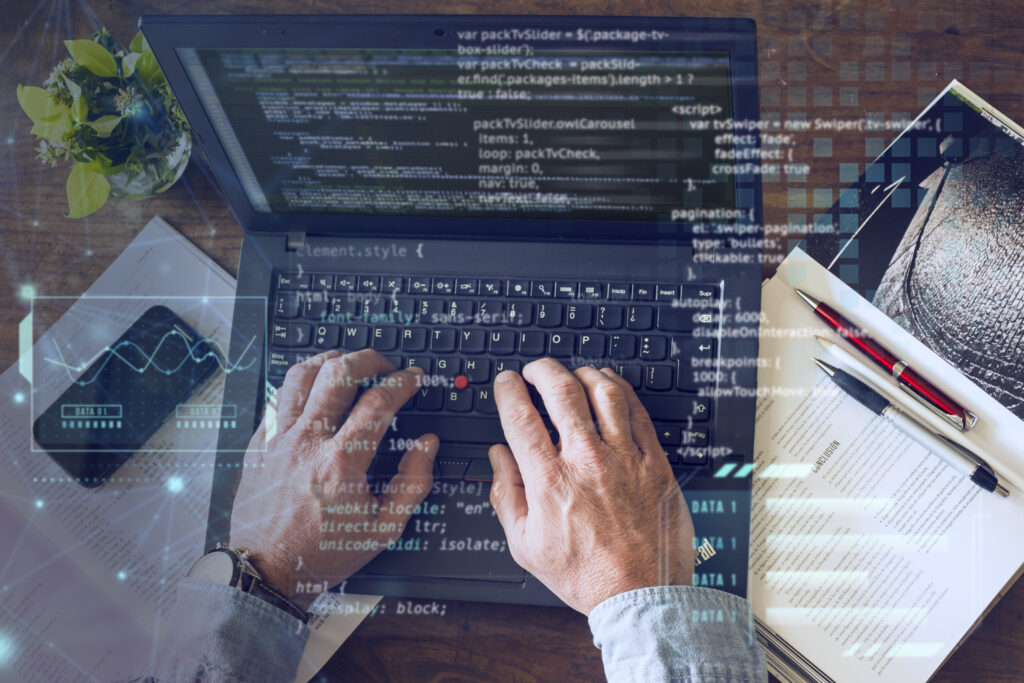
Why Choose Us?
- Expert Trainers: Learn from experienced professionals with in-depth knowledge of C/C++ and real-world development expertise.
- Comprehensive Curriculum: Gain a strong foundation in C/C++ programming, covering core concepts, advanced features, and practical applications.
- Hands-On Training: Work on real-world projects to build problem-solving skills and practical coding experience.
- Flexible Learning Options: Choose from flexible schedules, including weekend and evening batches, to fit your availability.
- Placement Assistance: Benefit from our career support, connecting you with leading companies for job opportunities.
- State-of-the-Art Facilities: Access modern infrastructure and tools to enhance your learning experience.
- Industry-Recognized Certification: Earn a certification that validates your expertise and boosts your professional profile.
- Global Alumni Network: Join a thriving network of successful professionals who started their careers with AdviTech IT Services.
Course Content
Data Structure
1. Arrays and Strings [4 hours]
2. Algorithm Analysis [3 hours]
- Time Complexity Analysis (Big O notation)
- Space Complexity Analysis
- Asymptotic Analysis of Algorithms
- Recursion and Recursive Algorithms.
- Practical
3. Linear Data Structure [9 hours]
- Stacks : Operations and Applications [theory/practical]
- Queue : Operations and Applications [theory/practical]
- Circular Queue : Operations and Applications [theory/practical]
- Deque : Operations and Applications [theory/practical]
- Practical
4. Linked Lists [7 hours]
- Operation – Creation, Insertion, Deletion [theory/practical]
- Doubly Linked Lists [theory/practical]
- Circular Linked Lists [theory/practical]
- Practical
5. Sorting Algorithm [5 hours]
- Bubble Sort [1 hour theory/practical]
- Selection Sort [1 hour theory/practical]
- Insertion Sort [1 hour theory/practical]
- Merge Sort [1 hour theory/practical]
- Quick Sort [1 hour theory/practical]
- Practical
6. Searching Algorithm [2 hours]
- Linear Search [1 hour theory/practical]
- Binary Search [1 hour theory/practical]
- Practical
7. Trees [11 hours]
- Binary Trees [theory/practical]
- Operation : Insert, Delete
- Traversal : Preorder, Inorder, Postorder
- Binary Search Trees [theory/practical]
- Operation : Insert, Delete
- Traversal : Preorder, Inorder, Postorder
- AVL Trees [theory/practical]
- Heaps [4 hours]
- Min heap
- Max heap
- Practical
8. Graphs I : Representation an Traversal [4 hours]
- Representation : Adancency Matrix, Adancency List
- Traversal : Breadth-First Search (BFS), Depth-First Search (DFS)
9. Graphs II : Basic Algorithms [4 hours]
- Minimum Spanning Tree Algorithms : Kruskal’s algorithm for minimum spanning tree,Prim’s algorithm for minimum spanning tree
- Shortest Path Algorithms : Dijkstra’s algorithm for shortest paths, Bellman-Ford algorithm for shortest paths
10. Tables [3 hours]
- Hashing Techniques
11. Sets [3 hours]
- Representation
- Operation : Union and Find
12. String Algorithms [7 hours]
- String manipulation and processing
- Pattern matching algorithms : Naive, Rabin-Karp, Knuth-Morris-Pratt (KMP)
13. Program Development [2 hours]
- Program Specification
- Pre and Post Condition
- Program Documentation