C/C++
C/C++ Programming Mastery
C/C++ programming forms the backbone of modern software development, powering everything from operating systems to advanced applications. Mastering these languages equips you with the tools to write efficient, high-performance code and solve complex problems. With their versatility and widespread use across industries like gaming, finance, and embedded systems, C/C++ offers endless career opportunities. Whether you’re learning the basics or advancing to topics like pointers, memory management, and object-oriented programming, achieving C/C++ programming mastery positions you for success in the ever-evolving tech landscape.
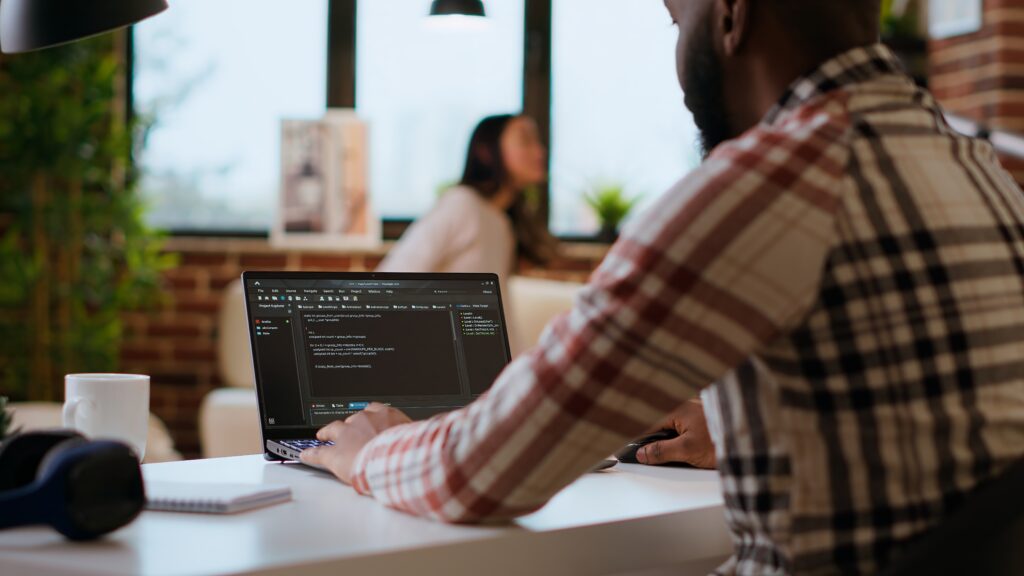
Why Choose Us?
- Expert Trainers: Learn from experienced professionals with in-depth knowledge of C/C++ and real-world development expertise.
- Comprehensive Curriculum: Gain a strong foundation in C/C++ programming, covering core concepts, advanced features, and practical applications.
- Hands-On Training: Work on real-world projects to build problem-solving skills and practical coding experience.
- Flexible Learning Options: Choose from flexible schedules, including weekend and evening batches, to fit your availability.
- Placement Assistance: Benefit from our career support, connecting you with leading companies for job opportunities.
- State-of-the-Art Facilities: Access modern infrastructure and tools to enhance your learning experience.
- Industry-Recognized Certification: Earn a certification that validates your expertise and boosts your professional profile.
- Global Alumni Network: Join a thriving network of successful professionals who started their careers with AdviTech IT Services.
Course Content
C Language
1. Introduction
- History of C language
- Memory structure of C language
- Compilers Used in C language
- Tokens used in C language
- What are Constant,Variables,Keywords
- Building first C program
- Process of compilation and execution of C program
- Details about error and debug programs
2. DataTypes
- Premetive datatypes
- Non premetive data types
- Premetive vs nonpremetives
3. Input and Output Instructions
- Need of control instructions
- Explaination on printf() and scanf()
4. Operators Used in C
- Arithematic operator
- Relationnal Operator
- Comparison Operator
- Conditional Operator
- Logical Operator
- Bitwise Operator
- Assignment Operator
- Increment/Decrement Operator
5. decision control instruction?
- If Statement
- If..else Statement
- Nested if Statement
- Ladder If statement
6. Looping Control Instructions
- For Loop Structure
- While Loop Structure
- Do While Structure
7. Case Structure
- Switch Structure
- Continue Structure
- Break Structure
- Continue vs Break Structure
- Default Structure
8. Functions In C Language
- What is a Function
- Need of Functions
- Function prototype
- Function Declaration
- Function calling
9. Storage Classes
- Automatic Storage
- External Storage
- Register Storage
- Static Storage
- Which to use and when
10. Array
- What is array
- Why to use an array
- One dimensional array
- Declaration of one dimensional array
- Memory structure of one dimensional array
- Initialization of one dimensional array
- Declaration of two dimensional array
- Memory structure of two dimensional array
- Initialization of two dimensional array
- Passing array element to function
- Passing entire array to function
- Disadvantages of array
11. Preprocessor
- Linker
- Loader
- Compiler
- Preprocessor
- Macros
- Working with macros
12. Pointer In C
- What is pointer
- Need of pointer
- Array vs pointer
- Types of pointer
- Calling function using pointer
- Various example using pointer
13. String Handling
- What is string
- Storing string
- Operation on string using array
- Operation on string using pointer
- Operation on string using in build function
14. Structure In C
- What is structure and its need
- Array vs structure
- Structure declaration
- Structure initialization
- Various operation on structure
15. File handling
- What is file and its need
- Opening a file
- Reading data from file
- Writing data on file
- Performing other file related operation
16. Dynamic Memory Allocation
- Usage of Malloc Calloc function
- Use of Free function
- Realloc, Alloc function
C++ Programming
1. Introduction
- History of C++
- Object Terminology
- C++ Characteristic
- Input/Output Services
2. Beginning with C++
- Structure of a C++ Program
- Using functions like cout(),cin()
- Principles of OOPS
- First C++ program
- Compilation and Execution process
3. Data Types
- Basic Data Types
- User Defined Data Types
- Derived Data Types
4. Classes & Objects
- How to make a Class and its Object
- Defining Data Members
- Defining Member Function
- Objects as Function Arguments
5. Functions in C++
- Function Delectration and Definition
- Variable Definition Del SCX
- Concept of Inline Function
- Default and Constant Aruments
- Function Overloading
6. Constructors & Destructors
- Basic Concept of Constructors
- Making a Constructor
- Types of Constructors
- Parameterized Constructor
- Copy Constructor
- Concept of Destructor
7. Operator Overloading
- Need of Operator Overloading
- Overloading Binary Operator
- Overloading Unary Operator
- Overloading Assignment Operator
8. Inheritance
- Concept of Inheritance
- Types of Inheritance
- Making Single Inheritance
- Multiple and Multilevel Inheritance
- Hybrid Inheritance
- Virtual and Friend Function
9. Pointers & Polymorphism
- Pointers to Objects
- This Pointer Pure
- virtual Functions
10. Storage Management in C++
- Memory Allocation in C++
- Dynamic Allocation:new delete
11. Exception Handling
- Basic of Error Handling
- Exception Handling Mechanism
- Catching Mechanism
12. Generic Templates
- Generic Template/Generic Function
- Concept Generic Classes
13. File Handling in C++
- Need of File Handling in C++
- Opening and closing a file
- File Pointer
- Updating a File Random Access
14. STL (Standard Template Library)
- Container
- Algorithm
- Linkedlist
15. Nested Classes in C++
- Need of Nested Classes
- Building and Working of Nested classes
16. Friend Function in C++
- What is Friend Function
- Operator overloding Friend Function